How to list directory files in Symfony
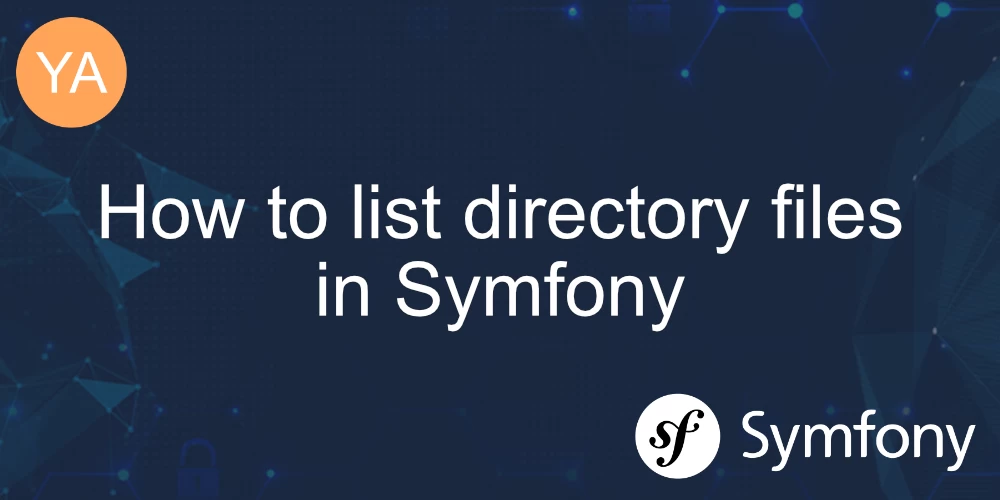
To list files in a directory with Symfony, you can use the Finder Component - $finder->files()->in(__DIR__);
Let's look at the example below to understand better how the Finder component works.
Symfony list files in a directory example
<?php
use Symfony\Component\Finder\Finder;
$finder = new Finder();
$finder->files()->in('my_directory');
foreach ($finder as $file) {
$fileNameWithExtension = $file->getRelativePathname();
echo sprintf('File %s found in directory', $fileNameWithExtension);
}
The example will list all files with their filenames in my_directory
.
Read file contents
You can read the file contents with $file->getContents()
.
// ..
foreach ($finder as $file) {
echo 'Contents: ' . $file->getContents();
}
List files by extension
You can filter directory files by extension, e.g:
// ..
$finder->files()->in('my_directory')->name(['*.php', '*.twig']);
foreach ($finder as $file) {
echo 'PHP or Twig file contents: ' . $file->getContents();
}
// ..
This example will filter files in my_directory
, which are .php
or .twig
files.
For more examples, you can check the official documentation.
What is Symfony Finder Component?
The Finder component offers a fluent and object-oriented API for searching, filtering, and working with files and directories. It's especially useful when you need to find files that match specific criteria, iterate through directories, or perform batch operations on files.