Symfony Twilio Notifier Example
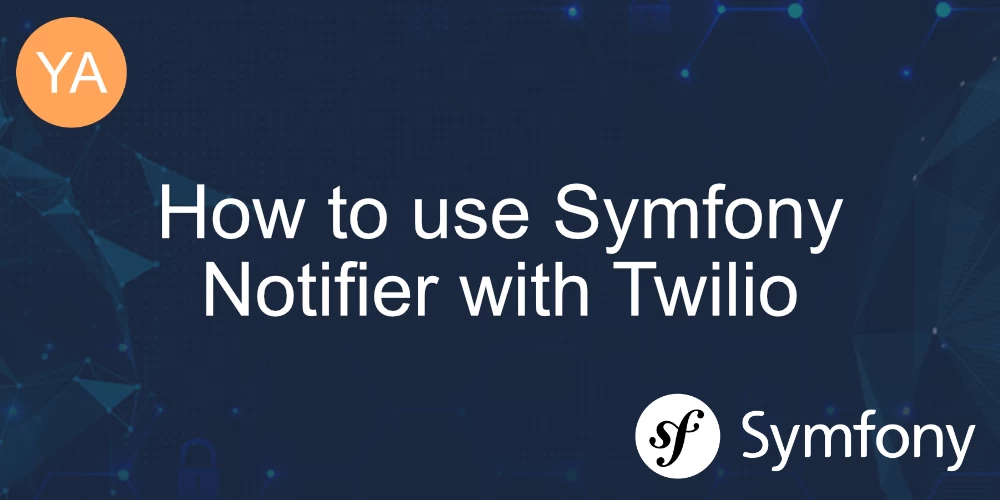
To use the Symfony Notifier component with Twilio, you need to install the symfony/twilio-notifier
package and configure it.
How to install Symfony Twilio Notifier?
To install the Twilio bridge run
composer require symfony/twilio-notifier
How to configure the Notifier component?
First, you need to enable the texter channel. Add the correct DSN to your .env
file
# .env
TWILIO_DSN=twilio://SID:TOKEN@default?from=FROM
Configure notifier package
# config/packages/notifier.yaml
framework:
notifier:
texter_transports:
twilio: '%env(TWILIO_DSN)%'
How to send SMS?
The TexterInterface allows you to send SMS messages.
// src/Controller/CheckoutController.php
namespace App\Controller;
use Symfony\Component\Notifier\Message\SmsMessage;
use Symfony\Component\Notifier\TexterInterface;
use Symfony\Component\Routing\Annotation\Route;
class CheckoutController
{
/**
* @Route("/checkout/success")
*/
public function checkoutSuccess(TexterInterface $texter)
{
$sms = new SmsMessage(
// the phone number to send the SMS message to
'+1411111111',
// the message
'Your order was successful!'
);
$sentMessage = $texter->send($sms);
// ...
}
}
The send()
method returns SentMessage
object which you may be helpful for debugging purposes.
$sentMessage = $texter->send($sms);
echo $sentMessage->getMessageId(); // fc603c57-c0df-40e3-a2b6-aba958395a01
What is Symfony Notifier?
The Symfony Notifier component simplifies the process of sending notifications by offering a consistent API to interact with different notification channels. Instead of dealing with the specifics of each notification channel's API separately, you can use the Notifier component to send notifications without having to rewrite code for each individual channel.
You can read more about Symfony Notifier in the official documentation.