How to use Symfony redirectToRoute with parameters
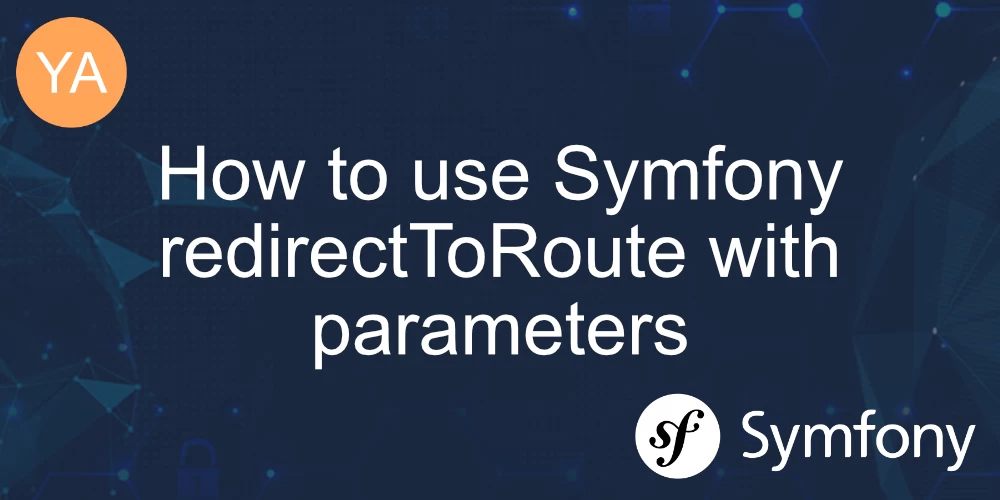
To use redirectToRoute
with parameters, pass the route name as the first argument and an array with parameters as the second argument of the method.
Here is an example of Symfony's redirect to route with parameters.
// ..
if ($form->isSubmitted() && $form->isValid()) {
// ..
return $this->redirectToRoute('admin.post.edit', [
'id' => $post->getId(),
]);
}
// ..
What does Symfony redirectToRoute
does?
In Symfony, the redirectToRoute
controller method is used to create a redirection response that directs the user's browser to a specific route within your application. This method is commonly used to perform URL redirection after a certain action has been taken, such as submitting a form or processing a request. The name of the method is essentially what it does - redirect to route.
Symfony redirectToRoute
with query parameters?
If you want to use redirectToRoute
with query parameters, you can to pass them in the second argument like the example above, Symfony will detect that they are not part of the route and will convert them to query parameters.
// .
public function view()
{
return $this->redirectToRoute('admin.post.edit', [
'id' => 5,
'customParameter' => 'hello',
]);
}
#[Route('/edit/{id}', name: 'admin.post.edit')]
public function edit(Post $post, Request $request)
{
// ..
}
// ..
The view()
method will redirect to /edit/5?customParameter=hello