What is the difference between let and var in Javascript?
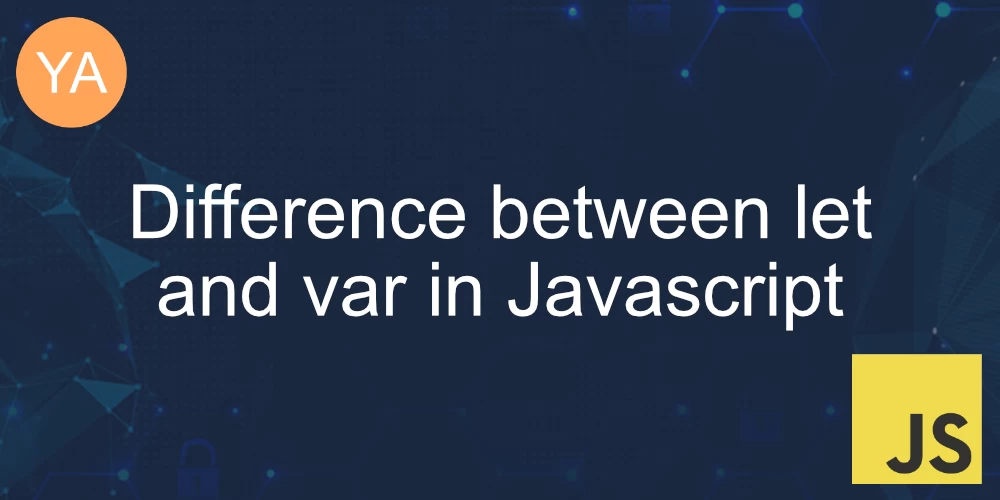
The difference between let
and var
is the scope in which the variables are available.
let
declares a block-scoped variable. They are accessible only within the block (enclosed by curly braces) in which they are defined.var
declares function scoped or globally-scoped variable. This means they are accessible throughout the entire function in which they are defined or globally if defined outside of any function.
But what does that mean? Let's look at the examples below to understand the differences better.
let
vs var
example
if (true) {
var varVariable = 'I am var';
let letVariable = 'I am let';
}
console.log(varVariable); // Outputs "I am var"
console.log(letVariable); // Throws "ReferenceError: letVariable is not defined"
varVariable
is globally available, whereas letVariable
is only available within the block {}
.
What is scope in JavaScript?
Scope in JavaScript refers to the context in which variables are defined and accessed. It determines where a variable can be used and where it is not accessible. Scopes can be global, function, or block-based, with variables having different levels of visibility within these scopes. The concept of scope helps manage the visibility and lifetime of variables, preventing unintended interactions and conflicts between different parts of the code.
Blocks only scope let
and const
declarations, but not var
declarations.
{
var myVar = 1;
}
console.log(myVar); // 1
{
let myLet = 1;
}
console.log(myLet); // ReferenceError: myLet is not defined
What is hoisting in JavaScript?
Hoisting in JavaScript is a behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase before the code is executed. This means that you can use variables and functions before they are actually declared in your code.
However, only the declarations are hoisted, not the initializations. This can sometimes lead to unexpected behavior and bugs if not understood correctly. It's important to be aware of hoisting to write more predictable and maintainable code.
var
hoisting
// using variable before it was declared
console.log(test); // undefined
var test;
As you can see in the example above, the test
variable is defined after console.log(test)
, but the no reference error is thrown. This is because var
declaration is initialized with undefined
before its actual declaration, potentially leading to unexpected behavior.
let
hoisting
// using variable before it was declared
console.log(test); // ReferenceError: Cannot access 'test' before initialization
let test;
we'll get
ReferenceError: Cannot access 'test' before initialization
Variable declaration
Variables declared with let
cannot be re-declared within the same scope, helping catch errors where you might accidentally declare the same variable name more than once.
{
let myLet = 1;
// do some work
let myLet = 2; // SyntaxError: Identifier 'myLet' has already been declared
}
where compared to var
{
var myVar = 1;
// do some work
var myVar = 2; // No problemo
}
Is it better to use let
instead of var
?
Yes, in most modern JavaScript development scenarios, it is generally recommended to use let
instead of var
.
Here are some reasons why let
is preferred over var
:
- Block Scoping: Variables declared with
let
have block scope. This helps prevent unintended variable leakage and makes the code more predictable and maintainable. - No Hoisting Issues: Variables declared with
let
are hoisted to the top of their block, but they are not initialized. This prevents the "hoisting" behavior ofvar
, where the variable is initialized withundefined
before its actual declaration, potentially leading to unexpected behavior. - Avoiding Global Namespace Pollution: Variables declared with
var
in the global scope become properties of the global object (e.g.,window
in browsers), which can lead to accidental global namespace pollution and potential conflicts with other variables. - Redeclaration Prevention: Variables declared with
let
cannot be re-declared within the same scope, helping catch errors where you might accidentally declare the same variable name more than once. - More Predictable Scoping: The use of
let
provides clearer scoping rules, which helps in understanding where a variable is accessible and where it's not. This contributes to code that is easier to read and maintain.
It's also a good practice to limit the scope of variables to the smallest context necessary for better code organization and to avoid potential issues. This helps in reducing the potential for bugs and makes it easier to reason about your code.
Conclusion
The choice between let
and var
in JavaScript revolves around the principles of modern coding practices and scoping clarity.
While both are used for variable declaration, let
is favored for its block-scoping nature, making variables confined to specific blocks of code.
This improves predictability and reduces unintended variable leaks.
By using let
, developers can ensure cleaner, more maintainable code with fewer chances of naming conflicts and unexpected behaviors. Embracing let
aligns with the evolution of JavaScript and best practices for coding clarity and predictability.
However, there are still cases where var
might be useful, especially if you're dealing with older codebases or specific scenarios where the hoisting behavior is desired.