How to delete doctrine entity by ID in Symfony
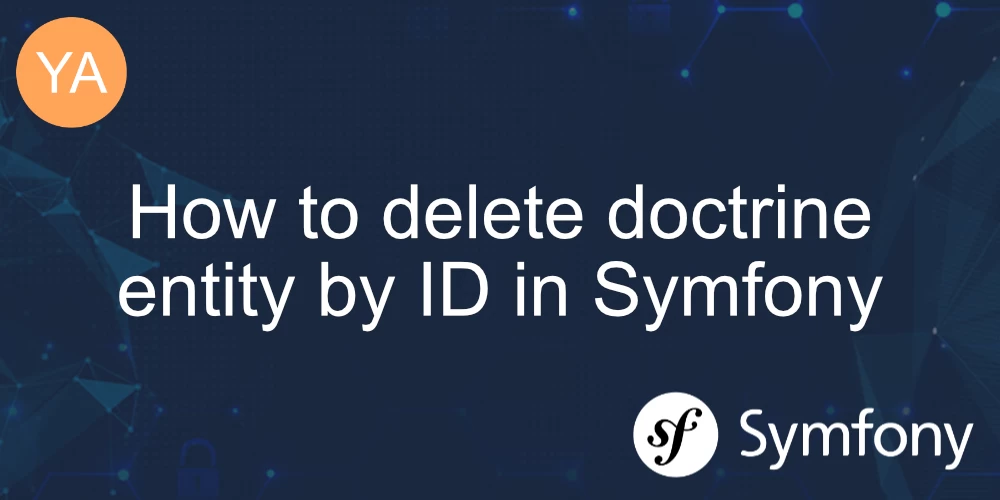
The easiest way to remove an entity in Doctrine ORM is by using the $entityManager->remove($entity)
method and then calling $entityManager->flush()
.
Here is a full Symfony/Doctrine example of entity removal
//..
#[Route('/remove/{id}', name: 'remove')]
public function removeAction(MyEntity $entity)
{
$em = $this->getDoctrine()->getManager();
$em->remove($entity);
$em->flush();
return $this->redirectToRoute('home');
}
//..
One very important thing. The DELETE SQL query is executed after calling $entityManager->flush()
.
Remove an entity without fetching it
You can use $entityManager->getReference()
to remove the entity without actually fetching it from the database. Let's see how that works:
//..
#[Route('/remove', name: 'remove')]
public function removeAction()
{
$em = $this->getDoctrine()->getManager();
$entityId = 5;
$em->remove($em->getReference(MyEntity::class, $entityId));
$em->flush();
return $this->redirectToRoute('home');
}
//..
The getReference
method is mainly used when referencing associations, but as you can see, it can also be used to remove things.
I suggest fetching the entity first and then removing it. That way, you validate that it exists, and fetching by ID is crazy fast, so the performance penalty is minimal.
Removing multiple entities
The most efficient way to remove multiple entities is to use a single DQL DELETE query.
Example:
<?php
$q = $em->createQuery('delete from App\Entity\Order o where o.processed = true');
$numDeleted = $q->execute();
Read more about Batch Processing.
Another way is to use $entityManager->remove()
, as shown in the first example.
Call $entityManager->remove($entity)
on each entity you want to remove, then call $entityManager->flush()
to execute the queries. Let's see how that looks:
//..
public function removeAction()
{
$em = $this->getDoctrine()->getManager();
$entityRepo = $em->getRepository(MyEntity::class);
$entity1 = $entityRepo->find(1);
$entity2 = $entityRepo->find(2);
$em->remove($entity1);
$em->remove($entity2);
$em->flush();
return $this->redirectToRoute('home');
}
//..
Be cautious about how many $entityManager->remove()
you stack before calling flush()
. It's a good practice to keep them low. For example, call $entityManager->flush()
for each 200 entity removals.