How to match URL with Symfony Router
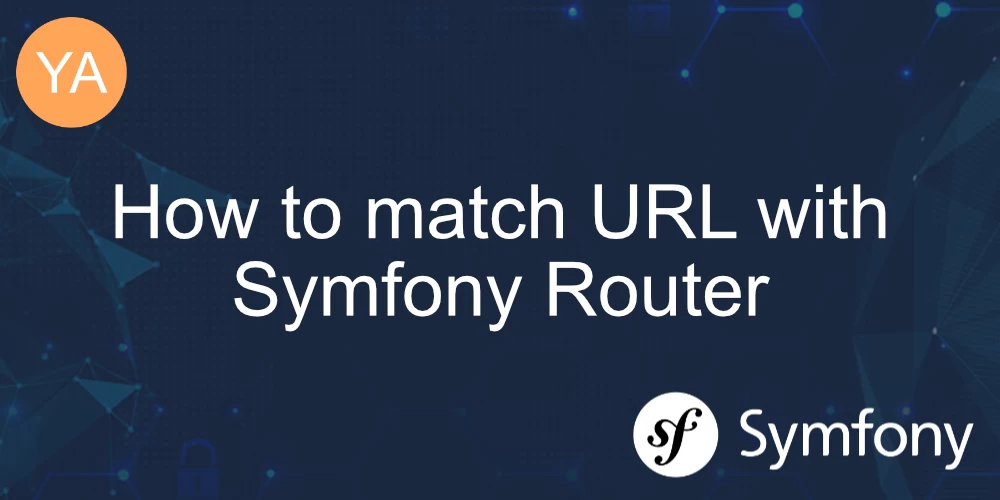
Have you ever needed to get a route name based on a given URL in Symfony? It might be a weird use case, but the RouterInterface
in Symfony has the match()
method that will help you do just that.
Let's see how match()
works:
#[Route('/test', name: 'test')]
public function test(RouterInterface $router)
{
dd($router->match('/say_hello/yoan'));
}
#[Route('/say_hello/{name}', name: 'say_hello')]
public function sayHello()
{
return $this->json([
'say' => 'hello',
]);
}
Opening /test
in my browser will output
HomeController.php on line 56:
array:3 [▼
"_route" => "say_hello"
"_controller" => "App\Controller\HomeController::sayHello"
"name" => "yoan"
]
Few things to note here.
Match() works only with relative URLs. If you pass an absolute URL - it will throw Symfony\Component\Routing\Exception\ResourceNotFoundException
.
The method will return an array with the route name and a controller name and method. Additionally, if the route has any parameters it will return them as well. Quite handy!
If you are wondering what is that mysterious function dd()
- it's part of the VarDumper component, which is designed to help you extract the state of any PHP variable.
This helper function also exists in Twig, but it's called dump()
and it can be used like this:
{{ dump(something) }}
It's basically var_dump() on steroids because it provides better visuals and it's interactive - if you have a huge entity or array the process won't just die, but it will present nice output.
Here is a dump of the pagination
variable in my Symfony Pagination example.