How to return JSON response from Symfony Controller
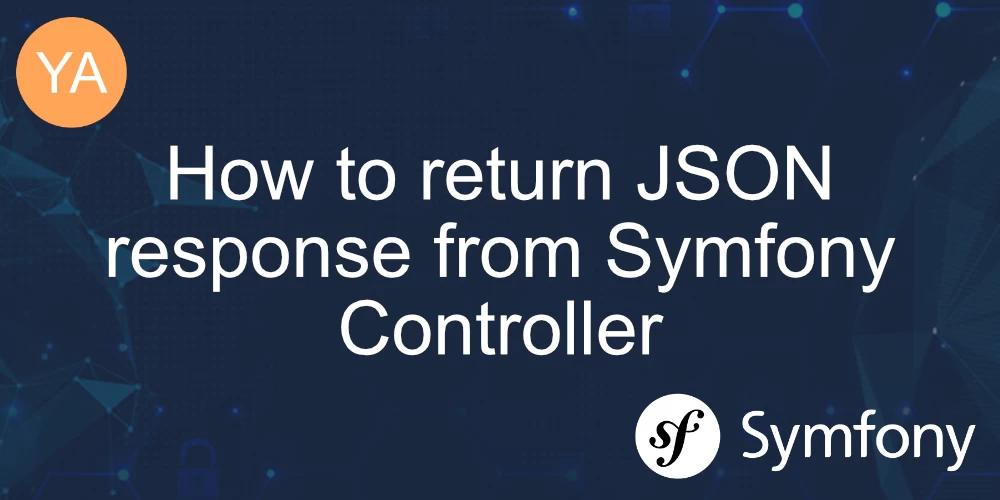
To return JSON response from Symfony Controller, you can use the $this->json();
method or new JsonResponse(['...']);
JSON (JavaScript Object Notation) is a lightweight data interchange format commonly used for transmitting and storing structured data. It consists of key-value pairs and arrays, making it versatile for representing various data types in a human-readable format that can be easily processed by computers. It is widely used in programming and especially in APIs.
Symfony return JSON response from controller
Symfony offers a few ways to return JSON response objects from a controller method.
Using $this->json();
// ..
use Symfony\Component\HttpFoundation\JsonResponse;
public function index(): JsonResponse
{
// Returns '{"website":"yarnaudov.com"}' and sets Content-Type: application/json
return $this->json(['website' => 'yarnaudov.com']);
// The shortcut defines three optional arguments
// return $this->json($data, $status = 200, $headers = [], $context = []);
}
// ..
$this->json()
is shortcut to new JsonResponse()
.
Using new JsonResponse()
// ..
use Symfony\Component\HttpFoundation\JsonResponse;
public function index(): JsonResponse
{
// Returns '{"website":"yarnaudov.com"}' and sets Content-Type: application/json
return new JsonResponse(['website' => 'yarnaudov.com']);
// The shortcut defines three optional arguments
return new JsonResponse($data, $status = 200, $headers = [], $context = []);
}
// ..
Return already formatted JSON as a response?
No problem. You can use the JsonResponse::fromJsonString()
static method for that which create JSON response from JSON formatted data.
$response = JsonResponse::fromJsonString('{ "data": 123 }');
Return entity as JSON
Symfony serializer components make it really easy to return entity as JSON.
Entity example
<?php
namespace App\Entity;
class Car
{
private string $model;
private int $year;
public function getModel(): string { return $this->model; }
public function setModel(string $model): void { $this->model = $model; }
public function getYear(): int { return $this->year; }
public function setYear(int $year): void { $this->year = $year; }
}
Symfony serializer JSON example in controller
// ..
#[Route('/serializer_test', name: 'serializer_test')]
public function serializerTest(SerializerInterface $serializer): JsonResponse
{
$car = (new Car)
->setModel('Enzo')
->setYear(2002);
return $this->json($serializer->serialize($car, 'json'));
}
// ..
will return
{
"model": "Enzo",
"year": 2002
}