How to run ElasticSearch queries with PHP and cURL example
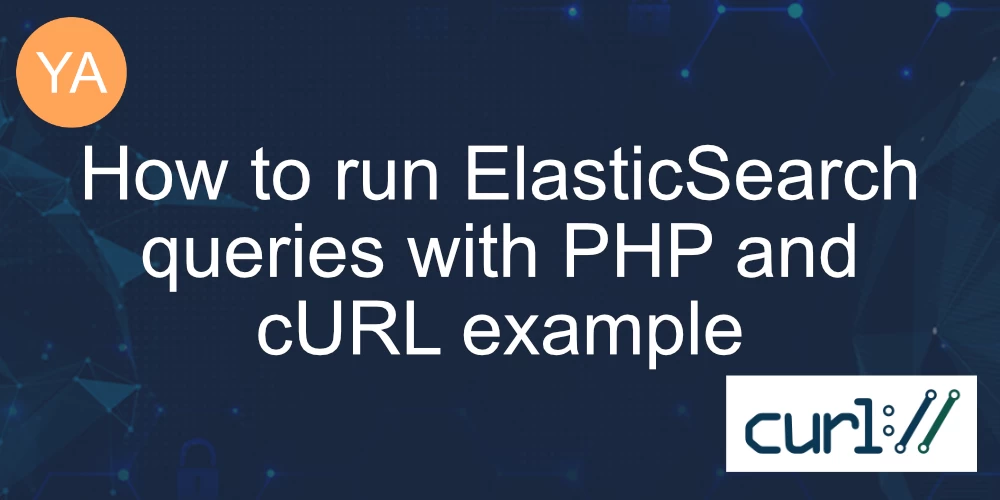
In the realm of modern data handling, Elasticsearch has emerged as a powerhouse, offering rapid data search and analysis.
As a PHP developer, integrating this robust search engine into your projects can elevate your data operations to new heights. In this article, we'll illustrate a concise example of how to create an index, create a document, get a document, and search for documents using PHP's cURL library.
The following examples will empower you to efficiently store, retrieve, and manipulate data through Elasticsearch's RESTful API, all within the familiar PHP environment.
PHP cURL ElasticSearch examples
Create index
Elasticsearch's Create Index API lets developers form new data containers called indexes. You can define index settings, mappings, and configurations through a basic HTTP request. This step sets the foundation for organized data storage, optimized searches, and scalability in your application. Let's see how we can create an index using PHP and cURL.
// Create index
$parameters = [
'mappings' => [
'properties' => [
'firstName' => ['type' => 'keyword'],
'lastName' => ['type' => 'keyword'],
'age' => ['type' => 'integer'],
],
],
];
$indexName = 'users';
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, "http://localhost:9200/".$indexName);
curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "PUT");
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($parameters));
curl_setopt($curl, CURLOPT_HTTPHEADER, ["Content-Type: application/json"]);
$res = curl_exec($curl);
curl_close($curl);
$response = json_decode($res, true);
var_dump($response);
returns
array(3) {
["acknowledged"]=>
bool(true)
["shards_acknowledged"]=>
bool(true)
["index"]=>
string(5) "users"
}
Create document
To create a document in ElasticSearch, you should specify the index name and pass the fields you want to add. Let's see an example of creating a document with PHP and cURL.
// Create document
$parameters = [
'firstName' => 'Yoan',
'lastName' => 'Arnaudov',
'age' => 32,
];
$indexName = 'users';
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, sprintf('http://localhost:9200/%s/_doc/', $indexName));
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($parameters));
curl_setopt($curl, CURLOPT_HTTPHEADER, ["Content-Type: application/json"]);
$res = curl_exec($curl);
curl_close($curl);
$response = json_decode($res, true);
var_dump($response);
outputs
array(8) {
["_index"]=>
string(5) "users"
["_type"]=>
string(4) "_doc"
["_id"]=>
string(20) "0aPMNooB9hSXXQIbAC2E"
["_version"]=>
int(1)
["result"]=>
string(7) "created"
["_shards"]=>
array(3) {
["total"]=>
int(2)
["successful"]=>
int(1)
["failed"]=>
int(0)
}
["_seq_no"]=>
int(0)
["_primary_term"]=>
int(1)
}
Get a document by ID
The Index API is used once again to get a document by ID.
$indexName = 'users';
$documentId = '0aPMNooB9hSXXQIbAC2E';
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, sprintf('http://localhost:9200/%s/_doc/%s', $indexName, $documentId));
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_HTTPHEADER, ["Content-Type: application/json"]);
$res = curl_exec($curl);
curl_close($curl);
$response = json_decode($res, true);
var_dump($response);
outputs
array(8) {
["_index"]=>
string(5) "users"
["_type"]=>
string(4) "_doc"
["_id"]=>
string(20) "0aPMNooB9hSXXQIbAC2E"
["_version"]=>
int(1)
["_seq_no"]=>
int(0)
["_primary_term"]=>
int(1)
["found"]=>
bool(true)
["_source"]=>
array(3) {
["firstName"]=>
string(4) "Yoan"
["lastName"]=>
string(8) "Arnaudov"
["age"]=>
int(32)
}
}
Search
To search for a document, you can utilize the the _search
endpoint. Let's see how you can search for documents in ElasticSearch using PHP and cURL.
$parameters = [
'query' => [
'query_string' => [
'query' => 'Yoan',
'default_field' => 'firstName',
],
],
];
$indexName = 'users';
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, sprintf("http://localhost:9200/%s/_search", $indexName));
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($parameters));
curl_setopt($curl, CURLOPT_HTTPHEADER, ["Content-Type: application/json"]);
$res = curl_exec($curl);
curl_close($curl);
$response = json_decode($res, true);
var_dump($response['hits']['hits']);
outputs
array(1) {
[0]=>
array(5) {
["_index"]=>
string(5) "users"
["_type"]=>
string(4) "_doc"
["_id"]=>
string(20) "0aPMNooB9hSXXQIbAC2E"
["_score"]=>
float(0.2876821)
["_source"]=>
array(3) {
["firstName"]=>
string(4) "Yoan"
["lastName"]=>
string(8) "Arnaudov"
["age"]=>
int(32)
}
}
}
Conclusion
PHP cURL library is powerful enough to be used for ElasticSearch integration. As seen in the PHP cURL ElasticSearch examples above, you can easily create indexes, create documents, search for documents, etc.
However, keep in mind that Elasticsearch queries can get more complex, involving JSON-formatted request bodies, different HTTP methods, and various query parameters. For more sophisticated use cases, consider utilizing a dedicated Elasticsearch library for PHP, such as the official Elasticsearch PHP client or other third-party libraries, as they provide higher-level abstractions and better error handling.