How to use Symfony process component with examples
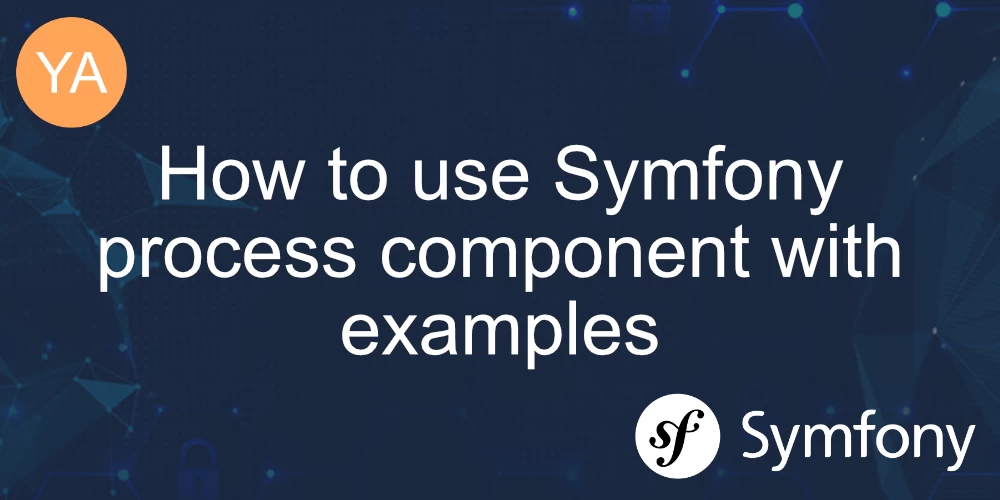
To use Symfony process, you need first to install the component:
$ composer require symfony/process
Then, you can run your first command
Symfony process example
use Symfony\Component\Process\Exception\ProcessFailedException;
use Symfony\Component\Process\Process;
$process = new Process(['ls', '-lsa']);
$process->run();
// executes after the command finishes
if (!$process->isSuccessful()) {
throw new ProcessFailedException($process);
}
echo $process->getOutput();
In this example, the code runs the ls -la
command, captures its output, and then prints the output to the console.
What is Symfony process component, and what is it used for?
The Symfony Process component is a part of the Symfony PHP framework that provides a convenient way to execute and manage external processes from within your PHP applications. It abstracts the underlying system-level functions and provides a more user-friendly and consistent interface for working with processes.
Symfony process in Laravel
You can use the Symfony Process component within the Laravel framework.
Laravel is built on top of many Symfony components, and it's designed to be highly modular and extensible. This means you can take advantage of Symfony components, including the Process component, within your Laravel applications.
Running a command without waiting for the process to finish
You can use the Symfony Process component to start a process without waiting for it to finish. This functionality is useful when you want to execute a process asynchronously, allowing your application to continue running while the external process operates in the background.
To start a process without waiting for it to finish using the Symfony Process component, you can use the start()
method instead of the run()
method. Here's an example:
use Symfony\Component\Process\Process;
$process = new Process(['php', 'path/to/your_script.php']);
$process->start();
In this example, the start()
method initiates the process execution and immediately returns control to your application, allowing it to continue running. The external process (php path/to/your_script.php
in this case) runs independently in the background.
Keep in mind a few important points:
-
Accessing Output: If you need to access the output or error stream of the process, you can still use the
getOutput()
andgetErrorOutput()
methods. However, since the process is running asynchronously, you might want to capture or log this output for later analysis. -
Process Control: You can use the
isRunning()
method to check if the process is still running, and you can also use thestop()
method to terminate the process if needed. -
Callbacks: The Process component allows you to set callbacks to execute when there's data available on the output or error streams. This can be useful for logging or monitoring purposes.
-
Exit Codes: Since you're not waiting for the process to finish, you won't be able to immediately retrieve the exit code. You can check the exit code later using the
getExitCode()
method once the process has finished. -
Concurrency and Resource Management: Be cautious about starting too many asynchronous processes simultaneously, as it could lead to resource contention and performance issues on your server.
Using the Symfony Process component to start processes asynchronously is particularly useful for scenarios where you want to offload time-consuming tasks, like generating reports or processing data, to background processes without blocking the main application's flow.
Will the child process terminate if the parent is killed/stopped?
If the parent process that started a child process is killed, the behavior of the child process depends on the operating system and the way the child process was launched.
-
Linux/Unix-like Systems:
On Linux and Unix-like systems, when a parent process is terminated, any child processes that were started by the parent process become orphaned. Orphaned processes are automatically adopted by the system's init process (usually with process ID 1). This means that even if the parent process is killed, the child process will continue to run until it completes its execution or is explicitly terminated.
However, the child process might not have access to the resources or context it was expecting from the parent process. This can lead to unexpected behavior or errors if the child relies on resources owned by the parent process.
-
Windows:
On Windows, the behavior can vary depending on how the child process was created and your Windows version. In some cases, killing the parent process might lead to the termination of the child process as well. In other cases, the child process might continue running even after the parent process is terminated.
If the child process was created using the Symfony Process component's
start()
method or a similar approach, the child process might continue running even if the parent process is killed.