How to use Symfony Query Builder count
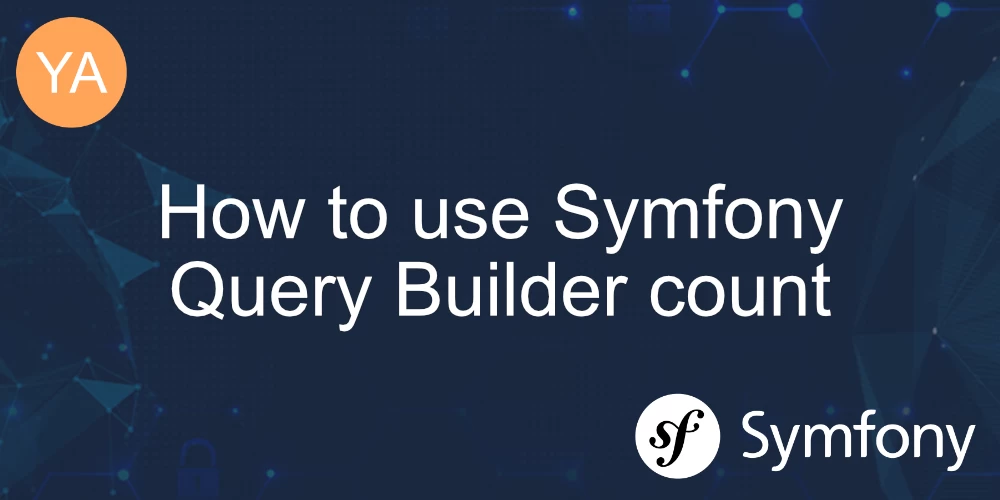
The Doctrine Query Builder in Symfony can be used to count rows by using ->select('count(e.id) as c')
Doctrine Query Builder count example
// ..
class PostRepository extends \Doctrine\ORM\EntityRepository
{
public function countReadPosts()
{
$res = $this->createQueryBuilder('p')
->select('count(p.id) as c')
->where('p.read = true')
->getQuery()
->getSingleScalarResult()
;
$readPostsCount = intval($res['c'] ?? 0);
}
// ..
By using select()
you can use standard SQL functions like count
to get the row count.
In the COUNT query example, we're using the getSingleScalarResult()
method to retrieve a single scalar result, which is the count of posts that match the specified condition.
Remember that the exact structure of your queries might vary depending on your entity's properties and your specific use case.