How to use Symfony Query Builder where
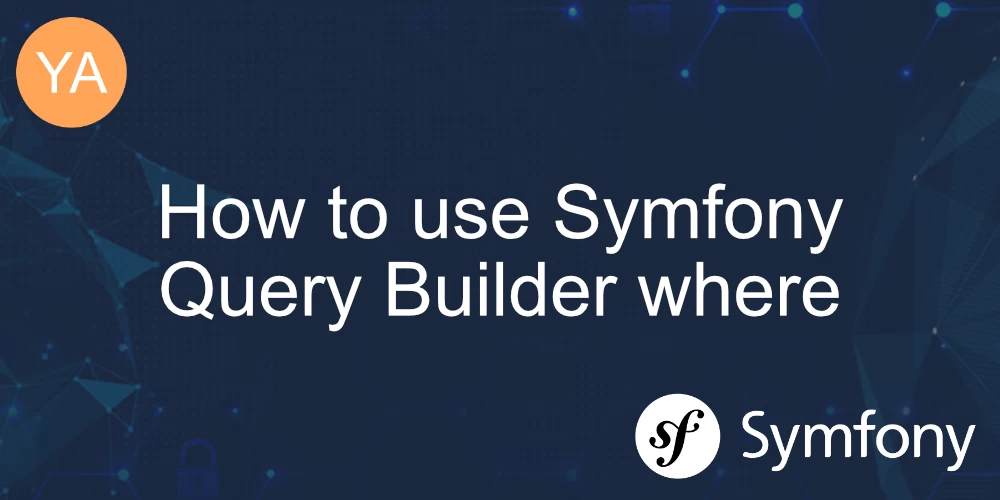
In Symfony, the Doctrine Query Builder provides a convenient and object-oriented way to construct complex SQL queries. The where()
method is used within the Query Builder to add conditions to your queries. Here's how you can use it:
Doctrine Query Builder where
example
// ..
class PostRepository extends \Doctrine\ORM\EntityRepository
{
public function getReadPosts()
{
return $this->createQueryBuilder('p')
->select('p')
->where('p.read = true')
->getQuery()
->getResult()
;
}
// ..
The example above will return only read posts. This Doctrine query raw SQL looks like this
select * from post where read = 1;
More complex examples with andWhere
and orWhere
You may need to add more where conditions, this is done with andWhere
and orWhere
.
Let's look at how to use them:
Doctrine Query Builder andWhere
example
// ..
return $this->createQueryBuilder('p')
->select('p')
->andWhere('p.read = true')
->andWhere('p.category = :categoryId')
->setParameter('categoryId', 5)
->getQuery()
->getResult()
;
// ..
Here we use andWhere
which always adds to the query.
Always use setParameter()
to add parameters to the query, that way to will prevent SQL injections, because setParameter
deals with that and escapes the values.
Doctrine Query Builder orWhere
example
In the Doctrine Query Builder, the orWhere()
method is used to add an additional OR condition to the WHERE clause of a query. This allows you to construct more complex conditions by combining multiple OR conditions within your query. Here's how you can use the orWhere()
method in Symfony with Doctrine Query Builder:
// ..
return $this->createQueryBuilder('p')
->select('p')
->orWhere('p.published = true')
->orWhere('p.read = true')
->getQuery()
->getResult()
;
// ..
This query will return entries which are p.published = true
or p.read = true
.
Multiple conditions in single where()
// ..
->where('p.published = true OR p.read = true')
// ..
is the same as
// ..
->orWhere('p.published = true')
->orWhere('p.read = true')
// .
The same is valid for andWhere()
.