How to use Symfony Query Builder delete
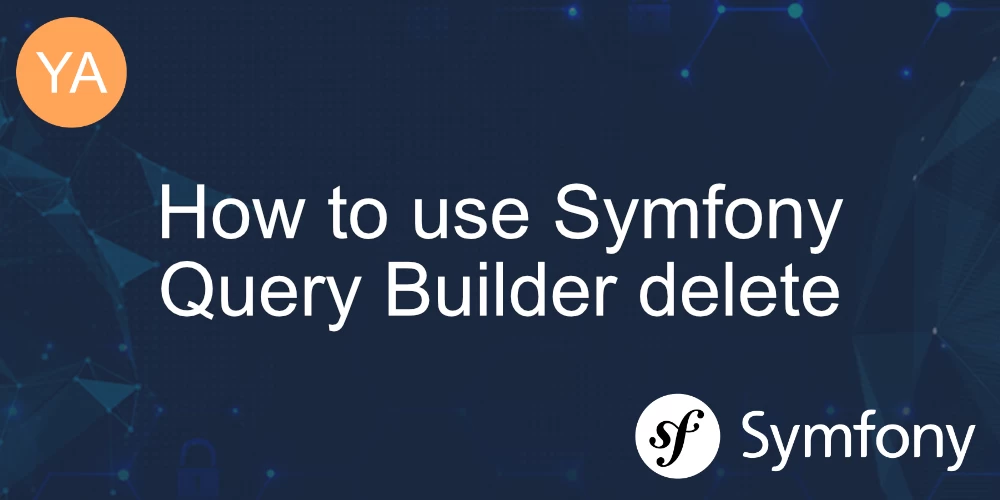
The Doctrine Query Builder in Symfony can be used not only to select entities but also to delete them by using ->delete()
.
Doctrine Query Builder delete example
The Doctrine Query Builder can also be used to build DELETE queries for removing records from a database table. Here's how you can use it to create a DELETE query in Symfony using Doctrine Query Builder:
// ..
class PostRepository extends \Doctrine\ORM\EntityRepository
{
public function deleteReadPosts()
{
$res = $this->createQueryBuilder('p')
->delete('App\\Entity\\Post', 'p')
->where('p.read = true')
->getQuery()
->execute()
;
}
// ..
In this example, we're using the delete()
method of the Query Builder to construct a DELETE query for the Post
entity. We're specifying the condition using the where()
method, which, in this case, deletes posts that are read.
After constructing the query, we call execute()
on the query to actually perform the deletion. The method returns the number of affected rows (i.e., the number of deleted products).
Remember to be cautious when using DELETE queries, as they directly impact your data. Always make sure to test thoroughly before performing mass deletions in a production environment.