How to use Symfony Query Builder update
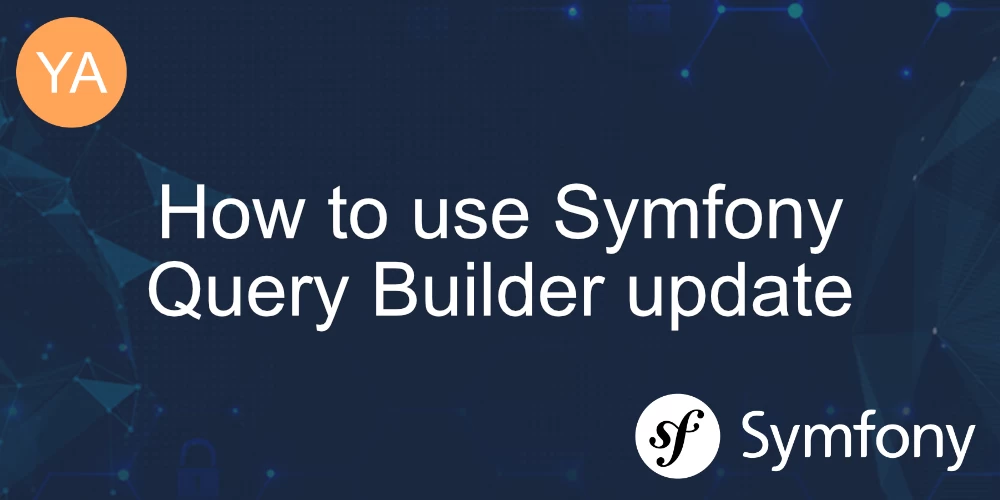
The Doctrine Query Builder in Symfony can be used not only for fetching entities but also to update them by using ->update()
.
Doctrine Query Builder Update example
// ..
class PostRepository extends \Doctrine\ORM\EntityRepository
{
public function updatePosts()
{
$qb = $this->createQueryBuilder('p')
->update('App\\Entity\\Post', 'p')
->set('p.read', true)
->where('p.read is null')
->getQuery()
->execute()
;
}
// ..
This code will update Posts
which are not read
and will set read=1
.
Remember to customize the query according to your specific use case. Be careful with updates, as they can significantly impact your data. Always test thoroughly before applying updates to production data.